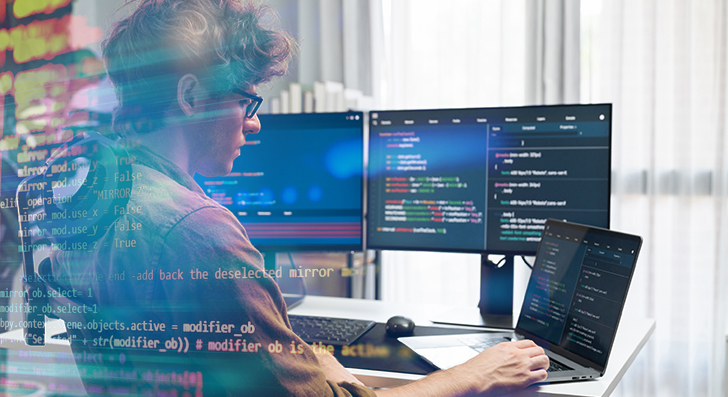
Scalability implies your software can tackle advancement—far more consumers, much more data, plus more targeted visitors—devoid of breaking. Like a developer, developing with scalability in your mind saves time and tension afterwards. Listed here’s a transparent and realistic guideline that may help you commence by Gustavo Woltmann.
Design for Scalability from the beginning
Scalability is not a little something you bolt on later on—it ought to be portion of the plan from the start. Many apps fail whenever they grow rapidly simply because the original style and design can’t deal with the additional load. As a developer, you must think early about how your procedure will behave under pressure.
Get started by coming up with your architecture to become versatile. Steer clear of monolithic codebases where all the things is tightly connected. Instead, use modular style or microservices. These patterns split your application into smaller, impartial pieces. Every single module or company can scale on its own devoid of influencing The complete method.
Also, contemplate your databases from day a single. Will it need to have to take care of one million customers or simply just a hundred? Choose the appropriate style—relational or NoSQL—according to how your info will improve. Approach for sharding, indexing, and backups early, even if you don’t require them nonetheless.
Another essential level is to stop hardcoding assumptions. Don’t produce code that only is effective less than current circumstances. Contemplate what would materialize if your user foundation doubled tomorrow. Would your application crash? Would the databases decelerate?
Use style and design designs that assist scaling, like concept queues or party-pushed devices. These enable your app handle much more requests with out obtaining overloaded.
Whenever you Make with scalability in your mind, you're not just preparing for success—you might be reducing upcoming problems. A very well-planned program is simpler to maintain, adapt, and grow. It’s better to arrange early than to rebuild later on.
Use the best Database
Choosing the right databases can be a essential A part of building scalable purposes. Not all databases are created the identical, and using the Erroneous one can slow you down or maybe induce failures as your app grows.
Start by being familiar with your knowledge. Is it really structured, like rows in the table? If Of course, a relational database like PostgreSQL or MySQL is a superb in good shape. These are typically robust with interactions, transactions, and consistency. In addition they help scaling techniques like read through replicas, indexing, and partitioning to handle additional site visitors and data.
Should your info is much more adaptable—like person activity logs, products catalogs, or documents—think about a NoSQL solution like MongoDB, Cassandra, or DynamoDB. NoSQL databases are much better at managing big volumes of unstructured or semi-structured facts and can scale horizontally additional effortlessly.
Also, look at your go through and produce designs. Are you undertaking lots of reads with less writes? Use caching and skim replicas. Are you dealing with a significant create load? Check into databases that can tackle substantial write throughput, as well as party-primarily based facts storage units like Apache Kafka (for short-term knowledge streams).
It’s also good to Consider ahead. You may not need to have Highly developed scaling functions now, but picking a databases that supports them indicates you gained’t will need to modify afterwards.
Use indexing to speed up queries. Prevent unnecessary joins. Normalize or denormalize your info based upon your obtain styles. And always keep track of database efficiency while you increase.
In short, the proper database depends upon your app’s structure, speed needs, and how you anticipate it to grow. Consider time to choose correctly—it’ll preserve a great deal of difficulties later on.
Enhance Code and Queries
Quick code is key to scalability. As your application grows, each individual compact delay adds up. Inadequately composed code or unoptimized queries can slow down overall performance and overload your program. That’s why it’s crucial to Develop efficient logic from the beginning.
Start off by composing thoroughly clean, simple code. Steer clear of repeating logic and take away nearly anything unneeded. Don’t select the most complex Alternative if a straightforward one particular functions. Keep the features brief, focused, and easy to check. Use profiling resources to find bottlenecks—sites the place your code requires much too prolonged to run or works by using excessive memory.
Subsequent, take a look at your databases queries. These usually slow factors down greater than the code alone. Ensure each query only asks for the info you really need. Stay clear of Pick *, which fetches every little thing, and instead decide on specific fields. Use indexes to hurry up lookups. And steer clear of accomplishing too many joins, In particular across massive tables.
If you observe a similar facts becoming asked for many times, use caching. Retailer the effects temporarily employing applications like Redis or Memcached so that you don’t really need to repeat highly-priced operations.
Also, batch your database functions whenever you can. As an alternative to updating a row one after the other, update them in groups. This cuts down on overhead and tends to make your app additional efficient.
Remember to examination with substantial datasets. Code and queries that function fantastic with one hundred records may well crash if they have to take care of one million.
In short, scalable apps are quickly apps. Maintain your code restricted, your queries lean, and use caching when wanted. These steps help your application continue to be smooth and responsive, even as the load raises.
Leverage Load Balancing and Caching
As your app grows, it has to deal with a lot more consumers plus much more targeted visitors. If all the things goes through one server, it'll rapidly turn into a bottleneck. That’s the place load balancing and caching can be found in. These two equipment support maintain your app quick, stable, and scalable.
Load balancing spreads incoming visitors across multiple servers. In lieu of one server accomplishing all of the work, the load balancer routes users to different servers dependant on availability. This suggests no solitary server gets overloaded. If one server goes down, the load balancer can mail visitors to the Other folks. Resources like Nginx, HAProxy, or cloud-based methods from AWS and Google Cloud make this very easy to build.
Caching is about storing knowledge briefly so it can be reused promptly. When end users request a similar facts once again—like an item site or even a profile—you don’t need to fetch it with the database each and every time. It is possible to serve it with the cache.
There are two popular forms of caching:
1. Server-aspect caching (like Redis or Memcached) shops facts in memory for quickly obtain.
2. Shopper-aspect caching (like browser caching or CDN caching) stores static documents near to the user.
Caching lowers database load, enhances velocity, and would make your app additional effective.
Use caching for things which don’t alter generally. And usually be certain your cache is up to date when details does transform.
In brief, load balancing and caching are uncomplicated but potent equipment. Together, they help your application handle a lot more buyers, stay rapidly, and Get better from issues. If you intend to mature, you will need each.
Use Cloud and Container Instruments
To build scalable programs, you would like tools that allow your application mature effortlessly. That’s the place cloud platforms and containers are available. They give you versatility, lessen set up time, and make scaling much smoother.
Cloud platforms like Amazon World wide web Providers (AWS), Google Cloud Platform (GCP), and Microsoft Azure Enable you to lease servers and products and services as you need them. You don’t need to get components or guess upcoming capacity. When site visitors will increase, you may increase extra resources with just a few clicks or automatically employing car-scaling. When targeted traffic drops, it is possible to scale down to save cash.
These platforms also offer you companies like managed databases, storage, load balancing, and protection instruments. You could deal with setting up your application as an alternative to taking care of infrastructure.
Containers are One more crucial Instrument. A container packages Developers blog your application and every thing it needs to operate—code, libraries, configurations—into one particular unit. This makes it easy to maneuver your app in between environments, from your notebook to your cloud, with no surprises. Docker is the most popular tool for this.
Once your app utilizes several containers, tools like Kubernetes assist you take care of them. Kubernetes handles deployment, scaling, and recovery. If 1 part within your app crashes, it restarts it immediately.
Containers also enable it to be very easy to independent aspects of your app into solutions. You could update or scale areas independently, that is perfect for functionality and trustworthiness.
In a nutshell, utilizing cloud and container applications implies you can scale rapid, deploy effortlessly, and Get better rapidly when complications take place. If you want your app to mature with no limits, commence applying these resources early. They help save time, decrease chance, and allow you to continue to be focused on constructing, not correcting.
Keep track of Anything
If you don’t keep an eye on your software, you won’t know when factors go Completely wrong. Monitoring aids the thing is how your application is performing, spot troubles early, and make improved decisions as your app grows. It’s a vital A part of constructing scalable units.
Commence by tracking primary metrics like CPU use, memory, disk space, and response time. These let you know how your servers and companies are accomplishing. Tools like Prometheus, Grafana, Datadog, or New Relic will help you acquire and visualize this knowledge.
Don’t just watch your servers—monitor your app too. Keep watch over just how long it requires for people to load webpages, how often problems come about, and wherever they come about. Logging instruments like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly may help you see what’s going on within your code.
Build alerts for significant challenges. Such as, In the event your reaction time goes earlier mentioned a Restrict or maybe a provider goes down, you must get notified quickly. This will help you correct concerns quick, normally right before people even detect.
Monitoring can also be beneficial whenever you make changes. If you deploy a completely new element and see a spike in errors or slowdowns, you could roll it back again just before it leads to serious problems.
As your app grows, traffic and facts boost. With out checking, you’ll overlook signs of trouble right until it’s way too late. But with the proper applications in position, you stay on top of things.
In short, checking assists you keep the app trusted and scalable. It’s not nearly recognizing failures—it’s about knowing your system and ensuring it really works properly, even stressed.
Ultimate Thoughts
Scalability isn’t just for significant firms. Even small apps have to have a powerful foundation. By planning carefully, optimizing correctly, and utilizing the proper applications, you'll be able to Establish apps that improve smoothly with no breaking stressed. Begin modest, think huge, and Make intelligent.